Spring 源码阅读:BeanDefinition 接口
前面说到 BeanDefinitionRegistry 是用来管理 BeanDefinition 的注册中心,但是还没看到 BeanDefinition 长什么样,下面就来了解下。
BeanDefinition 接口的继承结构
BeanDefinition 是一个接口,它用来描述 bean 实例,包括 bean 的属性值(property values)、作用范围(scope)、是否为 autowire 的首选(isPrimary)等。BeanDefinition 继承了两个接口,如下图所示
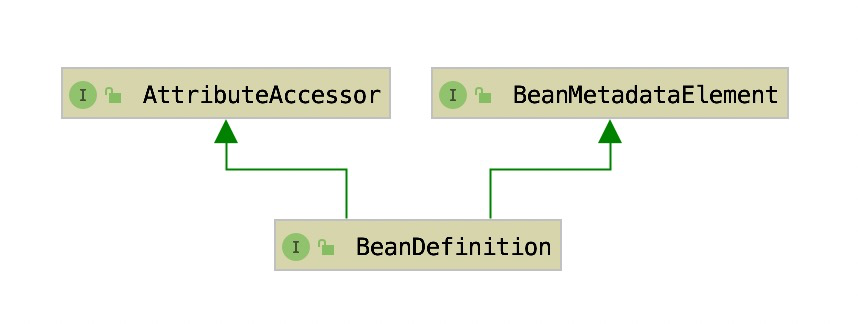
image-20200117003507554
下面先来了解下这两个接口
AttributeAccessor 接口
AttributeAccessor 定义了用于访问任意对象元数据的规范,它的方法定义如下:
1 | public interface AttributeAccessor { |
AttributeAccessorSupport 是该接口的一个实现,它的核心数据结构是一个 Map,所有的 「name -> 属性值」都保存在这个 Map 中
1 | /** |
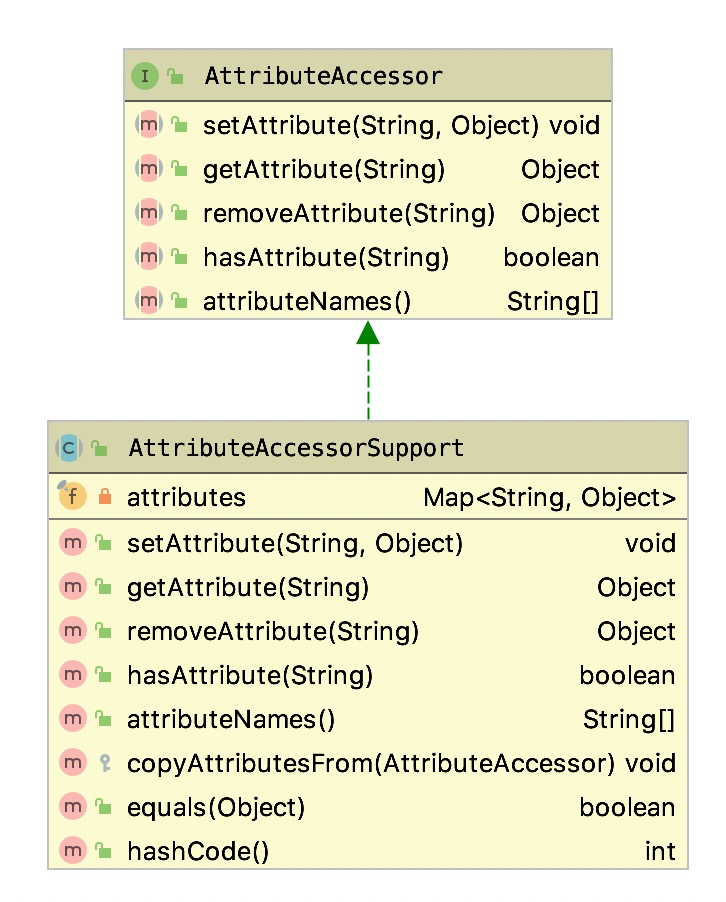
image-20200118002613747
这个类比较简单,主要看下 setAttribute 和 copyAttributesFrom 方法。setAttribute 的代码如下:
- 当 value 不为空时,直接添加到 Map 中
- 当 value 为空时,则将 name 对应的属性值从 Map 中移除
1 | public void setAttribute(String name, @Nullable Object value) { |
copyAttributesFrom 方法是留给子类使用的,它的作用是深度拷贝一个 AttributeAccessor
1 | protected void copyAttributesFrom(AttributeAccessor source) { |
BeanMetadataElement 接口
BeanMetadataElement 只有一个方法 getSource,用于获取 metadata element 对应的可配置的源对象
1 | public interface BeanMetadataElement { |
BeanMetadataAttribute 是 BeanMetadataElement 的一个简单实现。
作为 bean definition 的一部分,它用于持有一个键值对形式的属性,同时还会保存对应的源对象
除了保存 source 对象之外,BeanMetadataAttribute 还包含另外两个属性:name 和 value,用于保存属性名字和值:
1 | // 属性的名字 |
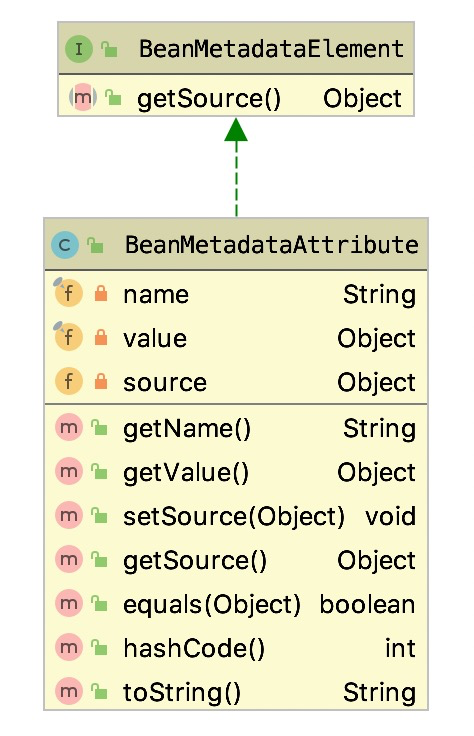
image-20200118002353955
BeanMetadataAttributeAccessor 实现
BeanMetadataAttributeAccessor 同时实现了 AttributeAccessor 和 BeanMetadataElement 两个接口,它的继承结构如下图所示:
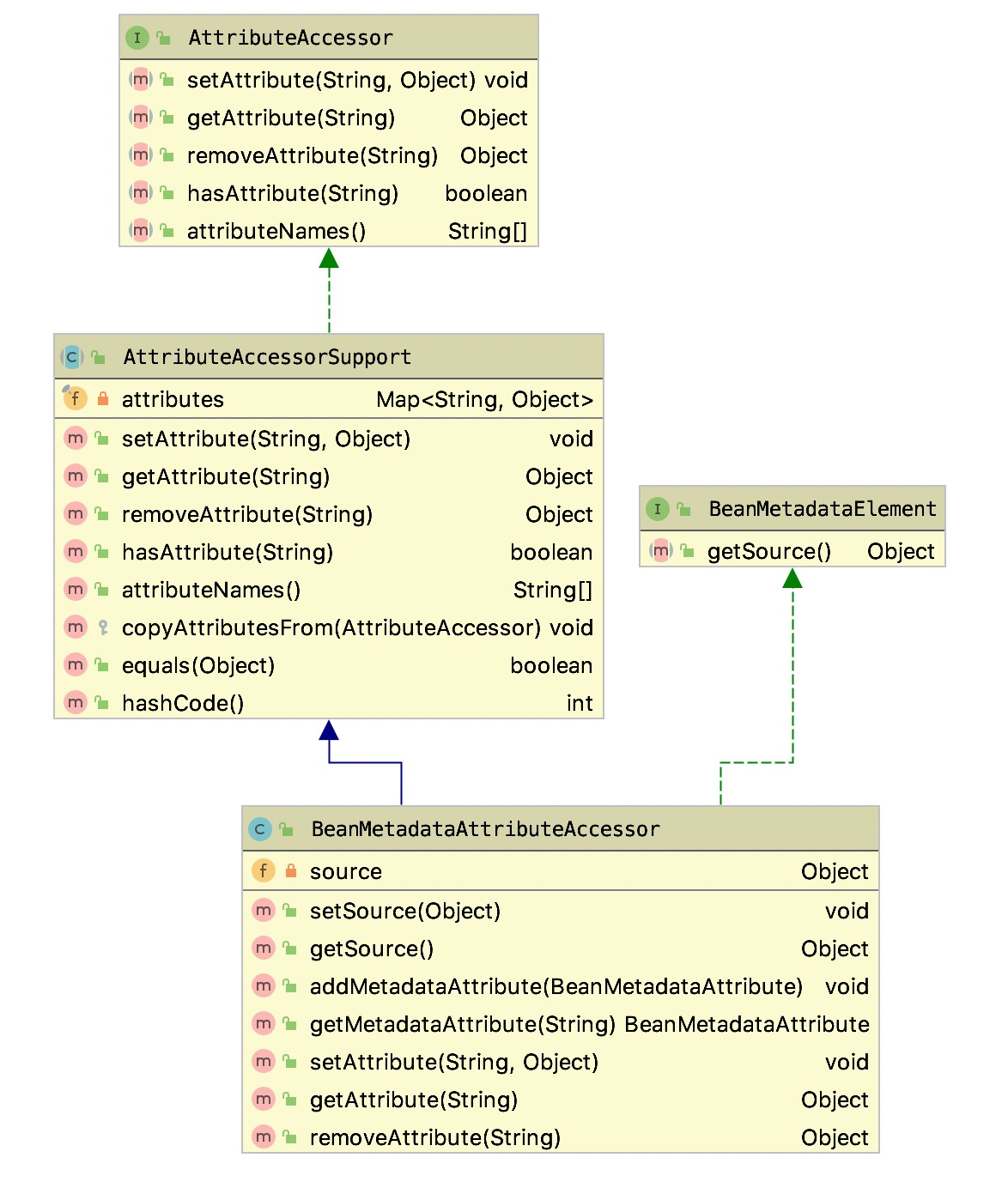
image-20200118003452774
可以看到,它继承了 AttributeAccessorSupport 类,可以使用其提供的方法来操作属性值,例如 addMetadataAttribute 方法直接调用 setAttribute 保存 BeanMetadataAttribute
1 | public void addMetadataAttribute(BeanMetadataAttribute attribute) { |
其他的 MetadataAttribute 操作实现类似。
它同时还实现了 BeanMetadataElement 接口,新增了属性
1 | private Object source |
同时提供了 get 和 set 方法
1 | public void setSource(@Nullable Object source) { |
BeanDefinition 接口的方法
BeanDefinition 用于描述 bean 实例,通常是根据 spring xml 中的 bean 配置生成的 BeanDefinition,当然也可以根据注解或其他源数据生成。BeanDefinition 中的方法可以参考 xml 中的 bean 配置来理解。
1 | public interface BeanDefinition extends AttributeAccessor, BeanMetadataElement { |
总结一下 BeanDefinition 的主要属性
属性 | 含义 |
---|---|
scope | 作用范围,例如 singleton、prototype 等 |
lazyInit | 是否延迟初始化 |
className | 对应的类的名字 |
parentName | 父级 bean definition 的名字 |
dependsOn | 依赖的 bean 的名字 |
autowireCandidate | 是否为 autowire 的候选者 |
primary | 是否为 autowire 的首选 |
factoryBeanName | factoryBean 的名字 |
factoryMethodName | 工厂方法的名字 |
initMethodName | 初始化方法的名字 |
destroyMethodName | 销毁方法的名字 |
ConstructorArgumentValues | 构造参数值 |
MutablePropertyValues | bean 实例的属性值 |
role | role 类型:APPLICATION、SUPPORT、INFRASTRUCTURE |
- 2020-01-14
BeanDefinitionRegistry 是一个接口,从名字上就可以看出来,它是一个管理 BeanDefinition 的注册中心。通常情况下,BeanFactory 的具体实现类也会实现该接口,用于管理 AbstractBeanDefinition 继承结构下的 BeanDefinition 实例,例如 RootBeanDefinition 和 ChildBeanDefinition 实例。
- 2020-01-19
BeanDefinition 用来描述 spring bean,是一个接口,它的具体实现类主要有 RootBeanDefinition、ChildBeanDefinition、GenericBeanDefinition 等,分别适用于不同的场景。
- 2020-01-22
XmlBeanFactory 可以说是 Spring 中一个最简单的 BeanFactory,通过它可以了解 Spring 的设计思路。
- 2020-03-31
当调用 BeanFactory.getBean 方法获取 Bean 时,如果 Bean 还未创建,则会触发 Bean 的创建,本文主要关注 Bean 的创建过程。
- 2020-04-29
PropertyPlaceholderConfigurer
用于加载指定的配置文件,替换 bean 配置中的占位符为指定的属性值。
预览: